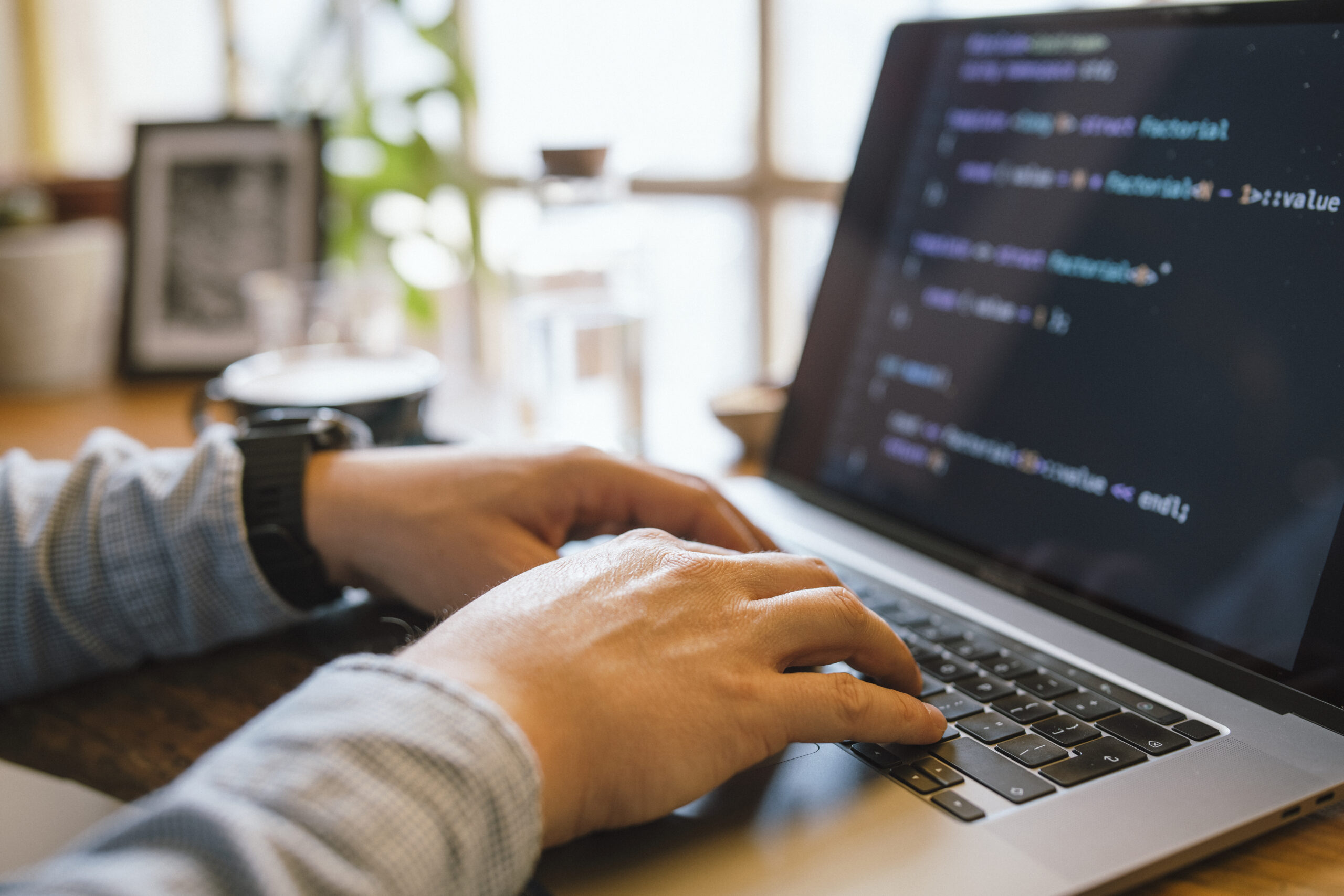
Debugging is Among the most necessary — however usually forgotten — competencies in a developer’s toolkit. It isn't nearly fixing broken code; it’s about comprehension how and why factors go Erroneous, and Discovering to Imagine methodically to unravel complications competently. Whether you're a starter or a seasoned developer, sharpening your debugging skills can help you save several hours of irritation and radically help your efficiency. Here's various approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Component of advancement, understanding how to connect with it properly throughout execution is Similarly critical. Modern day development environments appear equipped with impressive debugging abilities — but numerous developers only scratch the area of what these instruments can do.
Acquire, by way of example, an Integrated Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code around the fly. When made use of appropriately, they Permit you to observe accurately how your code behaves in the course of execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclude builders. They permit you to inspect the DOM, watch network requests, look at serious-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-amount builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory management. Understanding these equipment can have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Command systems like Git to be aware of code record, find the exact second bugs ended up released, and isolate problematic adjustments.
In the long run, mastering your applications means going past default options and shortcuts — it’s about producing an personal expertise in your improvement setting to make sure that when concerns come up, you’re not dropped in the dead of night. The greater you are aware of your resources, the more time you may shell out resolving the particular trouble rather than fumbling through the method.
Reproduce the trouble
The most significant — and infrequently missed — steps in efficient debugging is reproducing the trouble. Prior to jumping into the code or making guesses, developers require to create a dependable ecosystem or circumstance in which the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of opportunity, often resulting in wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire thoughts like: What steps resulted in The difficulty? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it will become to isolate the exact ailments less than which the bug occurs.
As you’ve collected more than enough details, try to recreate the challenge in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, look at creating automatic checks that replicate the edge situations or point out transitions involved. These assessments don't just assist expose the challenge but also avoid regressions Sooner or later.
Sometimes, the issue could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or underneath particular configurations. Making use of equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, plus a methodical technique. But when you finally can continuously recreate the bug, you're already halfway to fixing it. With a reproducible scenario, You should use your debugging resources a lot more properly, examination likely fixes safely and securely, and converse far more Obviously along with your group or consumers. It turns an abstract complaint right into a concrete challenge — Which’s where by builders prosper.
Read through and Recognize the Error Messages
Error messages are often the most valuable clues a developer has when a little something goes Completely wrong. Rather then observing them as annoying interruptions, developers ought to learn to take care of error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when below time pressure, look at the very first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root bring about. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These questions can information your investigation and point you towards the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable patterns, and Mastering to acknowledge these can dramatically hasten your debugging approach.
Some faults are vague or generic, and in All those cases, it’s critical to look at the context by which the error transpired. Look at associated log entries, input values, and up to date improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, minimize debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more impressive tools in a developer’s debugging toolkit. When utilized successfully, it provides actual-time insights into how an application behaves, assisting you realize what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic facts through growth, Data for basic occasions (like successful get started-ups), Alert for likely problems that don’t break the applying, ERROR for actual problems, and Lethal once the method can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and slow down your system. Deal with essential occasions, point out variations, input/output values, and critical final decision points in the code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Using a perfectly-believed-out logging technique, you can reduce the time it will require to identify problems, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technological job—it's a kind of investigation. To correctly identify and resolve bugs, developers have to solution the procedure like a detective solving a mystery. This attitude will help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis result in.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect just as much applicable information and facts as you can without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent image of what’s taking place.
Following, kind hypotheses. Request oneself: What might be creating this behavior? Have any variations a short while ago been designed on the codebase? Has this concern occurred before less than very similar conditions? The objective is to slender down opportunities and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge inside a managed setting. Should you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay back near attention to modest particulars. Bugs normally conceal in the minimum expected spots—like a missing semicolon, an off-by-one error, or a race issue. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future troubles and assistance Other people recognize your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden concerns in advanced units.
Create Exams
Composing assessments is among the simplest methods to boost your debugging capabilities and Over-all development efficiency. Tests not just aid catch bugs early but in addition function a security Internet that provides you self-assurance when generating changes for your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Start with unit tests, which focus on individual functions or modules. These small, isolated checks can immediately reveal whether a specific bit of logic is working as expected. When a test fails, you straight away know where by to look, noticeably lessening enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after previously being fastened.
Following, integrate integration checks and conclusion-to-stop tests into your workflow. These assistance be sure that different elements of your software operate with each other effortlessly. They’re notably beneficial for catching bugs that occur in advanced programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Assume critically about your code. To check a function adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with By natural means potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. After the exam fails regularly, you may focus on repairing the bug and enjoy your test pass when The problem is fixed. This approach ensures that the exact same bug doesn’t return Down the road.
In brief, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—gazing your screen for hours, attempting Remedy soon after Resolution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your thoughts, minimize stress, and sometimes see The problem from a new perspective.
When you're as well close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be less efficient at trouble-resolving. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also enable avert burnout, Specifically during for a longer period debugging periods. Sitting before a display, mentally trapped, is not simply unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer way of thinking. You could suddenly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all around, stretch, or do anything unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in speedier and more effective debugging Eventually.
In short, using breaks will not be a sign of weak point—it’s a wise strategy. It provides your Mind House to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Learn From Every single Bug
Each individual bug you encounter is more than just A brief setback—It is really an opportunity to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each can train you a little something beneficial should you make time to replicate and review what went wrong.
Start by asking your self several essential inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code assessments, or logging? The responses generally expose blind places with your workflow or being familiar with and help you build much better coding habits going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you figured out. After a while, you’ll start to see patterns—recurring challenges or popular faults—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, assisting others steer clear of the identical problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your improvement journey. In spite of everything, a number of the most effective developers are not those who write best code, but those that repeatedly discover from their faults.
In the end, Just about every bug you repair provides a new layer to the talent set. So upcoming more info time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.